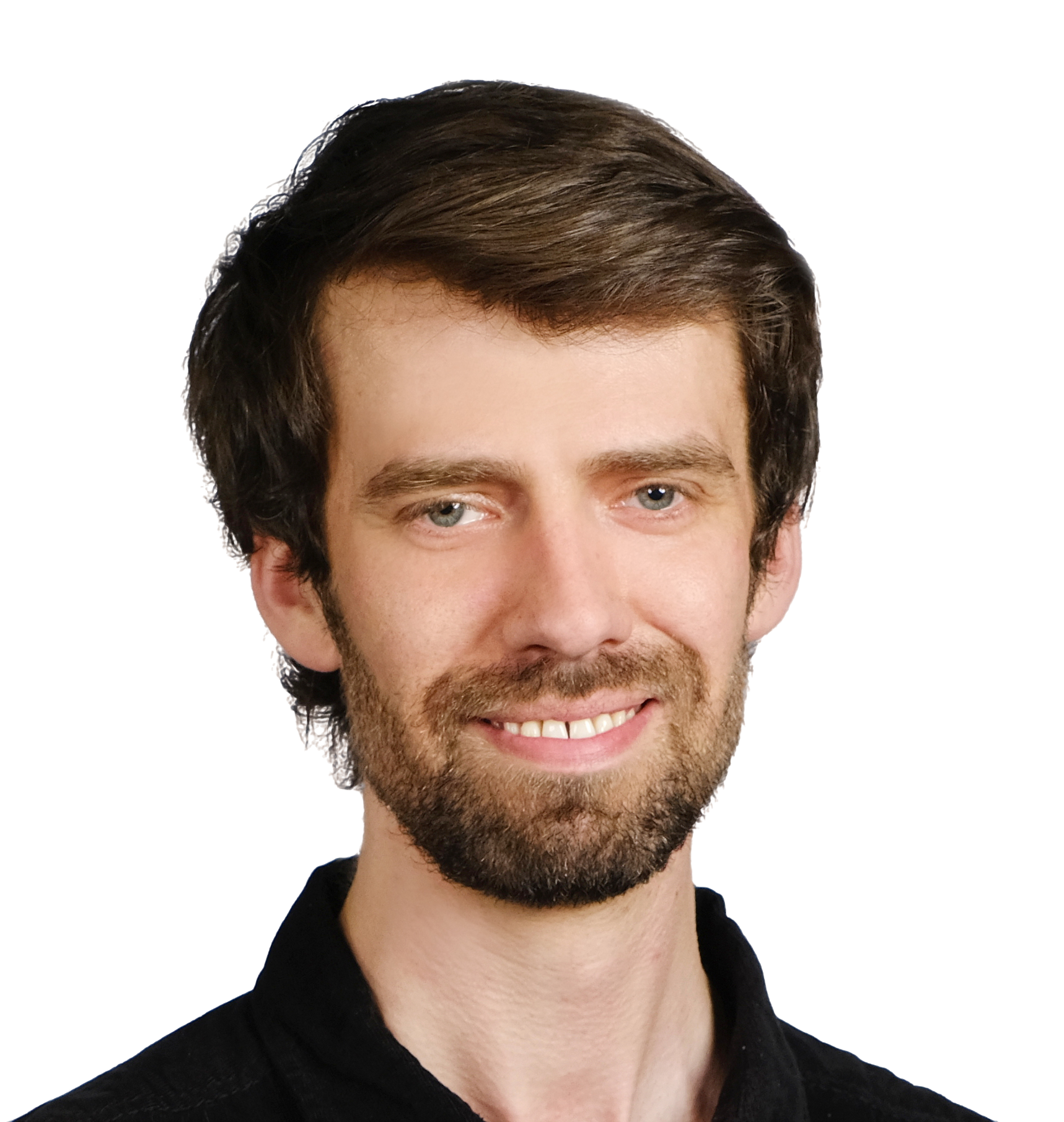
Mike McGrath
Fullstack Developer
Can I recursivley DFS a Binary Search Tree? Yes.
Can I vertically center a div? Maybe. Probably. What's the deadline again?
Technology
I Laravel
namespace App\Http\Controllers;
use App\Models\Todo;
use App\Http\Requests\StatelessStoreRequest;
class TodoController extends Controller {
public function store(StatelessStoreRequest $request){
return tap(Todo::create($request->safe()->all()), fn($todo) => response()->json($todo));
}
}
abstract class StatelessRequest extends FormRequest {
/**
* The Problem with:
* @see \Illuminate\Foundation\Http\FormRequest
*
* throw (new ValidationException($validator))
* ->errorBag($this->errorBag)
* ->redirectTo($this->getRedirectUrl());
*
* I mean, its called an "HttpFormRequest" object, so it's probably not fair to call it a "mistake"
* But either way, the hardcoded redirect method is assuming client state, and we don't do that here.
*
* @param Validator $validator
* @return array
*/
protected function failedValidation(Validator $validator)
{
throw new HttpResponseException(response()->json(['errors' => $validator->errors()], 422));
}
abstract public function rules();
}
View More Laravel Code Samples On Github
I AWS
export abstract class PHPDockerImageSource {
/**
* Uses a local image built from a Dockerfile in a local directory as the source.
*
* @param path - path to the directory containing your Dockerfile (not a path to a file)
*/
public static directory(path: string): PHPDockerImageSource {
return new PHPDockerAssetDirectorySource(path);
}
/**
* Bind grants the CodeBuild role permissions to pull from a repository if necessary.
* Bind should be invoked by the caller to get the SourceConfig.
*/
public abstract bind(scope: Construct, context: PHPDockerImageSourceContext): PHPDockerImageSourceConfig;
}
import * as cdk from 'aws-cdk-lib';
export class MyDockerImageDeployment extends cdk.Stack {
constructor(scope: Construct, id: string, props?: cdk.StackProps) {
super(scope, id, props);
const repository = new ecr.Repository(this, "phpbuilder", {
repositoryName: "phpbuilder"
});
const source = phpbuilder.PHPDockerImageSource.directory(path.join(process.cwd()));
const destination = phpbuilder.PHPDockerImageDestination.ecr(repository, {tag: 'latest'});
const image = new phpbuilder.PHPDockerImageBuilder(this, 'PHPBuilderStack', {
source,
destination
})
}
}
View More AWS & IAAC Example Code Samples On Github
FAQ
If you use the platform you should be able to find me easily. My link is: https://hired.com/x/715bf1f776370b039b78484e5b440846Feel free to email me a random leetcode problem with no explanation and no context. If you do, there's a very high chance I will get back to you with a solution within a day. That seems more fun. Or we could always whiteboard one together, either one.I love problem solving, and I respect the knowledge, but if I get a choice, I'm going to solve problems by building real things at this point in my career.
Do You Even Leetcode, Though?
I mean, it's not exactly my absolute favorite weekend activity, but if you asked me the time/space complexity of inserting into a linked list vs. a binary tree, or asked me to solve a leetcode'y style problem.. Yeah, there's a reasonably good chance I could do it.Hired.com gave me 10 weeks of profile promotion on their platform starting May 29th, 2023, so I have put the most of my leetcode'y time into their assessments as of today.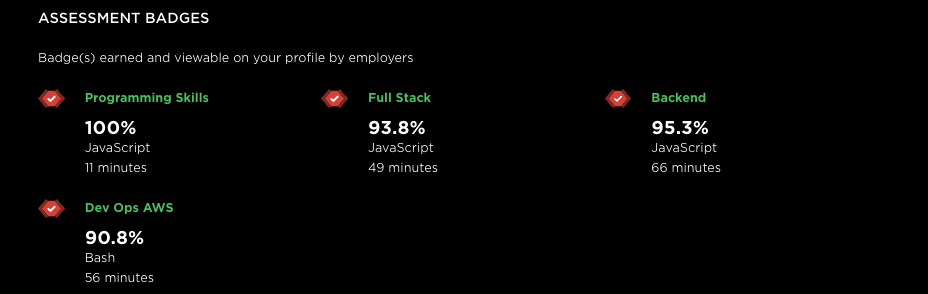